Selenium JavaScript and Mocha/Chai Framework Integration: Using Mocha and Chai for Test Automation with Selenium in JavaScript
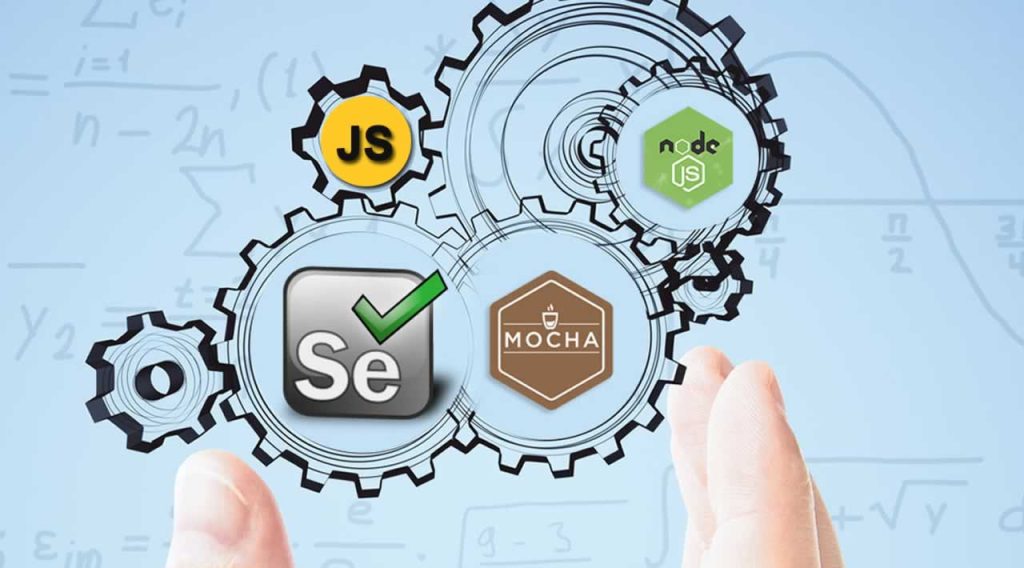
JavaScript is the most popular programming language. As web and mobile continue strengthening, JavaScript and JavaScript frameworks become even more widespread. So, it’s unsurprising that JavaScript has also become a top choice for test automation. Many recent advances have been made in open-source JavaScript test automation frameworks.
Now, we have several JavaScript testing frameworks that are robust enough for professional use. These scalable frameworks allow web developers and testers to automate unit tests and complete end-to-end test suites. Mocha is one such JavaScript testing framework.
Selenium also needs to be mentioned when discussing JavaScript test automation. It’s an essential tool for automation. So, In this article, we will explore how to use Selenium with JavaScript and integrate it with the Mocha and Chai frameworks for writing automated tests.
Table of Contents
Overview of Selenium
Selenium is an open-source test automation framework used for testing web applications. It supports browsers like Chrome, Firefox, IE, Safari, etc., and multiple languages like Java, C#, Python, JavaScript, Ruby, etc.
The components of Selenium are:
- Selenium Integrated Development Environment (IDE) – Used for developing tests in a recording and playback approach.
- Selenium WebDriver – It provides capabilities to automate testing by programmatically controlling a browser. It supports multiple languages like JavaScript, Python, C#, Ruby, etc.
- Selenium Grid – Distributes tests across multiple machines and executes them parallelly to reduce test execution time.
What is Mocha?
Mocha is a feature-rich JavaScript test framework that runs seamlessly on Node.js and browser environments. It provides functions to structure and organize tests familiarly. Below are some features of Mocha:
- Describe and It Blocks – The describe() function groups related tests together while it() defines test specifications. This provides a logical hierarchy and improved readability for tests.
- Asynchronous Support – Mocha fully supports testing asynchronous JavaScript code using async/await and promises without extra configurations.
- Flexible Interfaces – Mocha allows tests to be organized using BDD, TDD, or export interfaces to suit different styles.
- Parallel Execution – Tests can run concurrently using Mocha’s parallel mode to reduce overall execution time.
- Detailed Reporting – The default reporter outputs test results directly to the terminal. Additional built-in reporters, like spec, list, etc., provide more formatting and details.
- Browser Support – Mocha runs seamlessly in browser environments like JSDOM without extra configuration steps.
Mocha provides a flexible and fully-featured framework to handle all aspects of executing tests, including organization, running, reporting, and output.
What is Chai?
While Mocha provides the base framework, Chai is an assertion library that provides functions to test expected outcomes.
It supports three assertion styles:
- Expect – Enables writing assertions using a familiar BDD syntax like expect(result).to.equal(expected).
- Assert – Provides traditional assert style methods like assert.equal(result, expected).
- Should – Allows expressive assertions through chainable BDD methods like result.should.equal(expected).
What makes Mocha a robust JavaScript Testing Framework?
Mocha stands out among JavaScript testing frameworks for several reasons:
Installation Flexibility
Mocha is an adaptable JavaScript testing framework with flexible installation options. It can be installed globally on a machine to make it available for testing across different projects and codebases. This is ideal for developers working on multiple repositories.
Mocha can also be installed as a devDependency for a specific project. This bundles Mocha with that project for isolated testing needs.
Finally, Mocha enables browser-based testing by directly linking to the CDN. This is useful for testing front-end web apps and UIs now in the browser. Mocha can fit into any workflow or infrastructure needs with its global, local, and browser-based installation methods.
Cross-Browser Testing Capabilities
A key strength of Mocha is its cross-browser testing capabilities. Mocha tests run smoothly across all modern browsers, including Chrome, Firefox, Safari, and Edge. The Mocha team performs rigorous browser compatibility checks with each new release.
Updated JavaScript and CSS builds are included to ensure optimal performance across environments. Mocha also provides browser-specific methods and options so tests behave as expected in different browsers.
For example, Selenium Webdriver allows controlling test actions in the browser. Reporter colors can be adjusted for lighter or darker browser themes. With its robust cross-browser testing, Mocha enables developers to identify issues that only occur in specific browsers during the development process.
AI-powered test orchestration and execution platform like LambdaTest offers an online Selenium grid with access to over 3000+ real browser and operating system environments to enhance cross browser testing further. By integrating LambdaTest, Mocha automated tests can be run at scale across an extensive matrix of desktop and mobile browsers. This ensures comprehensive test coverage across modern browser versions, configurations, and platforms.
Customizable Reporting
Mocha offers flexible options for customizing test reports to match project needs. The default Mocha reporter displays test results in a readable hierarchical format ideal for scanning. Mocha also includes additional built-in reporters like list, progress, JSON, etc. You can choose the reporter that best captures the test data you need. Developers can build custom reporters using the Mocha reporter API.
With customizable reporting, you can generate output sound for their workflow. Reports can be integrated into CI/CD pipelines or display only the details needed for debugging. Mocha’s flexible reporting ensures practical test analysis.
Integration with Assertion Libraries
Mocha integrates smoothly with popular JavaScript assertion libraries like Chai, Should.js, Expect.js, and more. This integration removes the need to build custom assertions from scratch, reducing redundant code.
Developers can leverage common assertion patterns like matches, spies, and stubs within Mocha test cases, speeding up test writing. Integration with assertion libraries cuts down on testing overhead and effort. Mocha handles the test runner, while assertions validate behavior and results using clean, readable code.
Supports Both TDD and BDD
One aspect that makes Mocha unique is its support for both test-driven development (TDD) and behavior-driven development (BDD) workflows. Its simple test interface and integration with assertion libraries cater to TDD for writing isolated test cases individually.
On the other hand, Mocha enables BDD through its describe() and it() syntax popularized by frameworks like Jasmine and Cucumber. This provides an easy-to-read structure. Developers can implement either TDD or BDD with Mocha. They can even mix both approaches using TDD for unit tests and BDD for integration and end-to-end tests. Mocha provides the flexibility to choose the correct technique per test.
Synchronous and Asynchronous Testing
Mocha stands out by handling both synchronous and asynchronous testing scenarios. Callbacks allow it to manage asynchronous testing for non-blocking I/O operations, network calls, and other async behaviors. The async/await syntax makes asynchronous tests even cleaner. At the same time, Mocha enables synchronous testing by simply returning without callbacks, falling back to a synchronous style.
This flexibility supports diverse testing needs on small or large projects. Sync tests work great for simple functions and utilities. Meanwhile, async tests can spin up servers, query databases, or simulate complicated user flows. Mocha handles both synchronous and asynchronous testing with ease.
Getting Started with Mocha/Chai and Selenium in JavaScript
Step 1: Install Node JS and Node Package Manager (npm)
Mocha requires Node.js to be installed. If it isn’t installed, you can download the Windows Installer from the nodejs.org website or use the npm manager.
Once Node.js is installed, the npm package manager can install other modules like Mocha.
Step 2: Create a Project Folder
Open a terminal/command prompt and enter the following:
mkdir my-test-project cd my-test-project |
This will create a new folder called my-test-project and navigate into it.
Step 3: Initialize npm Project
Initialize a new npm project by running:
npm init -y |
This will generate a package.json file with default settings.
Step 4: Install Test Dependencies
Install Mocha, Chai, and Selenium WebDriver as dev dependencies:
npm install –save-dev mocha chai selenium-webdriver |
This will install and add the testing libraries to your package.json under devDependencies.
The next step would be to write some test scripts using Mocha and Selenium WebDriver and assertions with Chai.
Step 5: Creating Our First Mocha Test Script
Create a tests folder and add a Mocha test file basic.js:
// tests/basic.js const { assert } = require(“chai”); const { Builder, By } = require(“selenium-webdriver”); describe(“Google Search”, function() { let driver; before(async function() { driver = await new Builder().forBrowser(“chrome”).build(); }); after(async function() { await driver.quit(); }); it(“can search for Selenium”, async function() { await driver.get(“https://www.google.com”); await driver.findElement(By.name(“q”)).sendKeys(“Selenium”); await driver.findElement(By.name(“q”)).submit(); assert.include(await driver.getTitle(), “Selenium”); }); }); |
Step 6: Configure npm Script
In package.json, add the following test script:
“scripts”: { “test”: “mocha ‘./tests/*basic.js'” } |
Step 7: Run the Test
In the terminal, run:
npm test |
Now, Mocha will execute the test case and output the results.
Conclusion
Through this article, we have explored combining Selenium web automation with JavaScript, leveraging the Mocha test framework and Chai assertion library. By setting up the Selenium WebDriver API in Node.js, we can automate browser testing in the versatile JavaScript language. Mocha provides a structure for organizing test suites while maintaining readability. The expressive Chai assertions integrate cleanly to validate outcomes. Running tests locally or distributing them on Selenium Grid allows flexible execution. These tools create a robust end-to-end testing approach for complex modern web applications.
JavaScript developers now have an avenue to build durable test automation frameworks in a familiar programming environment. The journey starts by installing packages via npm, writing initial test specs, and expanding as needs evolve. This integrated stack delivers a scriptable, scalable solution through Selenium, Mocha, and Chai for those seeking to implement continuous testing practices in their JavaScript projects.