Getting Started with Selenium Python Automation: A Beginner’s Guide
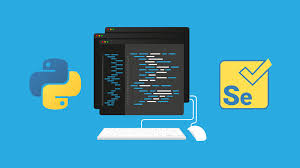
Selenium, along with Python, is a powerful and reliable combination used for automating web browser interactions. It provides a simple API to write functional tests using Selenium WebDriver. The prowess of Selenium and Python helps in automating interactions with WebElements in the DOM (Document Object Model).
While Selenium provides the framework, Python is the programming language, making the process intuitive and efficient. This combination is especially popular for web application testing and web scraping. This emphasizes on the framework’s strong standing in the testing community and shows the need for a dedicated resources and support system.
Now whether you are a beginner looking to explore the world of automation or an experienced programmer wanting to expand your Selenium testing skills, this beginner’s guide will assist you with an introduction to Selenium Python automation.
Table of Contents
Let us start with Selenium and its Automation Capabilities
Selenium was developed initially as a browser automation tool, but now it is an open source framework with a suite of tools and libraries which enables automation testing of web applications across different platforms and browsers. Years passed it has developed and has evolved into a platform which supports multiple programming languages, like it lets you write test scripts in programming languages like Ruby, Java, JavaScript, C#, PHP, and Perl.
It also provides many functions such as automating user interactions, navigating between the web pages, and validating web elements. One can easily simulate user actions, such as clicking buttons, filling out forms, and navigating through web pages. This allows the user to automate repetitive tasks and perform comprehensive testing efficiently, making Selenium a widely adopted tool in the software testing and quality assurance community.
Python for Selenium Automation and its benefits
Speaking of one of the most trusted, widely followed and beginner friendly programming languages is Python. It is readable and easy to use and a high-level interpreted programming language. It has a simple syntax and has a large and supportive community that makes it a best choice for running automation tasks. Many programming paradigms, including procedural, functional programming, and object-oriented are also supported by it.
It is easily readable and because of this using Python for Selenium automation becomes the most important feature. Test scripts are easy to write and maintain because of the clean and understandable code. Tests on any browser like Firefox, Chrome, IE, etc can be performed and the only thing changes are the WebDrivers, which are specific for each browser.
Setting Up the Environment for Selenium Python Automation
Here are the steps that must be followed to start working on Selenium Python automation as you first need to set up your development environment.
Installing Python: At first you must start by downloading and installing Python on your computer. On the official website that is phthon.org you will find the latest version of Python available. You can download it later and then follow the installation instructions to complete the process.
Installation of Selenium WebDriver: On completion of installation of Python, next you need to install the Selenium WebDriver library. Then open a command prompt or terminal and later run the following command: pip install selenium.
Installation of Web Browser Drivers: Selenium WebDriver then interacts with web browsers using specific driver executable files. Following this you have to download and install the appropriate driver for the browser you want to carry out automation testing. To understand this let us consider that if you want to automate Chrome, you simply need to download the ChromeDriver from the official Selenium website.
Hence by setting up the environment, you are now ready to start with Selenium Python automation.
First let’s get started with Selenium WebDriver
The key component for automating web browsers is Selenium WebDriver. Let us now explore how to set it up and perform basic tasks using Selenium WebDriver with Python.
Next installing Selenium WebDriver for Python: To install Selenium WebDriver for Python, you can use the following command: pip install selenium
The necessary packages required for Selenium WebDriver to work with Python will be downloaded and installed using this command.
Configuring Web Browsers for Selenium Testing
Upon installing Selenium WebDriver, next you need to configure it to work with specific web browsers. Let us understand this with an example: you are automating Chrome again; you need to download the ChromeDriver executable from the official Selenium website and then add its location to the system’s PATH environment variable. This allows Selenium to locate and use the ChromeDriver for automation.
Navigating and Interacting with Web Elements
You can start automating interaction with web elements when Selenium WebDriver is already set up. Some common tasks included are navigating to a web page, filling out forms, clicking buttons, and retrieving data from elements carried out.
To understand how to navigate to a web page, one can use the get() method of the WebDriver object, which provides the URL as an argument. For example: from selenium import webdriver
driver = webdriver.Chrome()
driver.get(‘https://www.example.com’)
To interact with web elements, one should use methods like find_element_by_*() to locate the elements and perform required actions. For example, to click a button:
button = driver.find_element_by_id(‘submit-button’)
button.click()
You can also leverage AI-powered test orchestration and execution platforms like LambdaTest, which offers a Selenium grid for automation testing. It allows you to run Selenium automation scripts on a cloud of 3000+ desktops and mobile environments, which helps eliminate the need to set up and maintain your own infrastructure.
Essential Selenium Python Automation Techniques
Now at this point being familiar with the basics of Selenium Python automation, let us dive into some essential techniques which will make the automation scripts more efficient and reliable.
Locating Web Elements using Different Strategies
The first step required is to locate web elements to start with the interaction.
Various methods provided by Selenium to locate the elements are as by the ID, by tag name, class name, CSS selector, or XPath.
Let us see it in example, if you want to locate an element with a specific ID:
element = driver.find_element_by_id(‘element-id’)
To ensure that the scripts works best across different scenarios it is important to choose a reliable and follow the unique locator strategy.
Performing Actions on Web Elements
On completion of locating the web element, various actions can be performed on it, such as typing text, clicking, or retrieving information. It provides methods like click(), send_keys(), and text property to perform these actions.
Let us consider an example, entering a text(‘Hello, World!’) into an input field:
input_field = driver.find_element_by_id(‘input-field’)
input_field.send_keys(‘Hello, World!’)
Handling Web Alerts, Frames, and Windows
There are different stages or times when the web application may encounter alerts, frames, or any multiple windows, in such cases Selenium provides methods to handle situations like these when they occur. Now let us have a look at how to interact with each of them one by one.
1) Dealing with alerts:
alert = driver.switch_to.alert
alert.accept() # Accept the alert
2) Switch to a frame:
frame = driver.find_element_by_id(‘frame-id’)
driver.switch_to.frame(frame)
3) Switch to a new window:
window = driver.window_handles[-1]
driver.switch_to.window(window)
Building Robust Selenium Python Tests
As we know Selenium Python is one of the most widely used automation testing tools. It is open-source and free to use. It ensures the reliability and effectiveness of your browsers test cases or web applications. It is very important to follow best practices for building robust test scripts. Now let us have a glance on how to build a strong Selenium Python Test.
Designing Effective Test Cases
A well designed test case is very important and crucial for proper test coverage. Every test case must focus on a specific function or scenario and it must be independent of any other test case. By doing so better maintenance is achieved and debugging is also done easily.
Implementing Assertions for Test Validation
To validate or check the behavior of an application and make sure that application is performing as per the expectation, Selenium provides methods like assertTrue() and assertEqual() for asserting the conditions.
For better understanding let us consider an example; to assert the title of a web page:
title = driver.title
self.assertEqual(title, ‘Expected Title’)
Organizing Test Suites and Executing Multiple Tests
It is very essential to organize your test cases into logical groups or test suites as the test suite grows eventually and by organizing it becomes easy in management and for test execution. Selenium is friendly with various frameworks and helps in organizing and executing test cases and these frameworks allow in running multiple tests in a controlled manner and provide a detailed report.
Working with Waits for Synchronization
Selenium provides a built-in wait mechanism to make sure that the automation test scripts wait for specific conditions every time before proceeding. As synchronization is very important aspect in automation as the web pages take time to load or even may appear or disappear dynamically.
Let us consider an example for the same; to wait for an element to be clickable:
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
wait = WebDriverWait(driver, 10)
element = wait.until(EC.element_to_be_clickable((By.ID, ‘element-id’)))
Managing Cookies and Handling Authentication
Small pieces of text sent to your browser by a website you visit, is called a cookie. Website applications often rely on these cookies for management and authentication. Now Selenium allows managing these cookies, deleting, adding or even modifying them.
Lets understand how to add a cookie;
cookie = {‘name’: ‘cookie-name’, ‘value’: ‘cookie-value’}
driver.add_cookie(cookie)
And for handling HTTP authentication, you can pass the username and password as part of the URL:
driver.get(‘http://username:password@example.com’)
Summary
In this blog, we understood the basics of Selenium Python automation. We learnt how Selenium allows automation testing of web applications and also the benefits of using Python for automation. Here we also discussed how to set up the environment for Selenium Python automation, how to get started with Selenium WebDriver, and also perform the important automation techniques. In conclusion by following this blog as a beginner’s guide, you can acquire the necessary skills to start automating tasks and perform comprehensive testing using Selenium WebDriver and Python.